Nested Loop
Similar to how conditionals can be nested, we can also nest loops. For example, this code below:
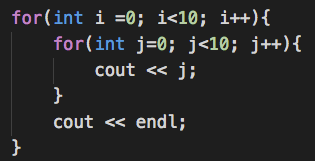
It will print the following:
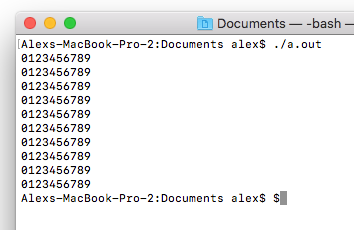
How did this work?
- The first for loop, lets call loop i, is executed first. So it executed all the codes within loop i.
- Within loop i is a second for loop, called loop j. Within loop j is a cout statment that just prints out the value of j.
- But the entire j loop must be ran before continuting. So it print all numbers of j, which is 0-9.
- Notice that all the numbers of j loop got printed and we are still in the first iteration of loop i.
- After j teminates, the program jumps back up to loop i, increments i, and executed loop j again.
- This process is done 10 times.
Pattern Printing
Printing patterns can be done with a nested loop. Below are examples of how to print patterns. In all of these examples, keep in mind that you must print an entire row before moving onto the next row. It really helps to think that the pattern is in a numbered grid.
Rectangles
Each iteration of the inner loop prints an X. The entire inner loop prints a row of COLS Xs. After you finish printing a row, then print a newline. Repeat the row/newline combination ROWS times. Notice that the newline is in the outer loop but not the inner loop.
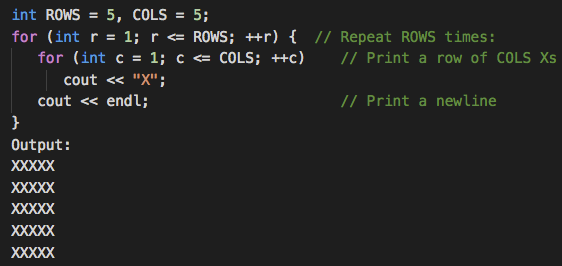
Non-solid rectangles
A rectangle where the character to print depends on a condition. To do this, replace the cout statement in the inner loop with a conditional statement. The condition will depend on the current row or column (the loop counters r and c), and may depend on the rectangle size. Imagine the pattern on a numbered grid.
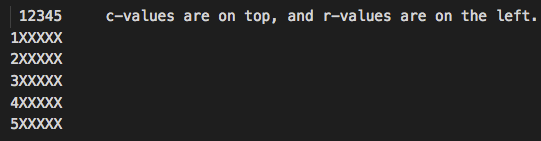
Example: Middle column Os
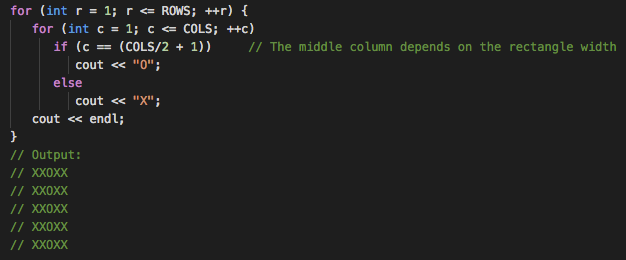
Example: Cross (middle column Os and middle row Os)
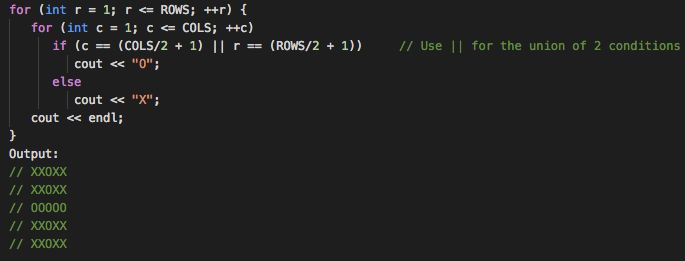
Example: O on even rows
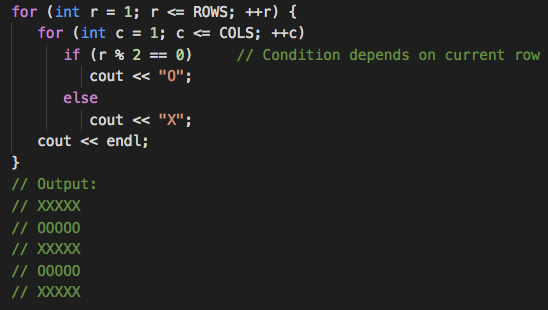
Squares with diagonal lines
When printing diagonal lines, it can help to think of the condition as a math function. In the function y = mx + b, m is the slope and b is the y-intercept. In the examples above we used r and c, so r==c is equivalent to the math function y=x (slope = 1, y-intercept = 0).
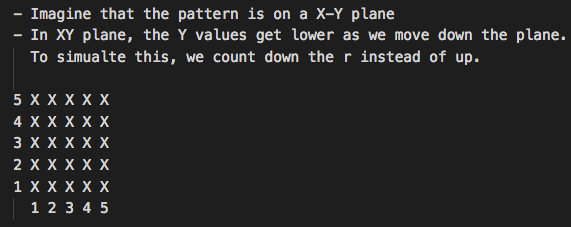
Example: Diagonal of Os
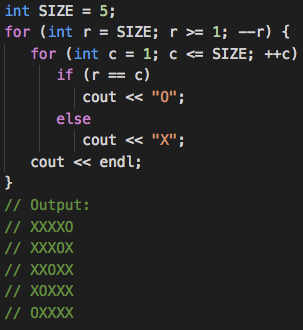
In this example, r counts down instead of up to coincide with the X-Y plane used in math (higher Y-values on top). Counting up would also work (but then the function would be different).
Example: Diagonal shifted up by 2
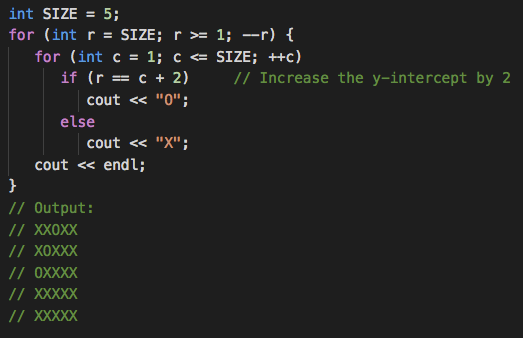
Example: Opposite-direction diagonal
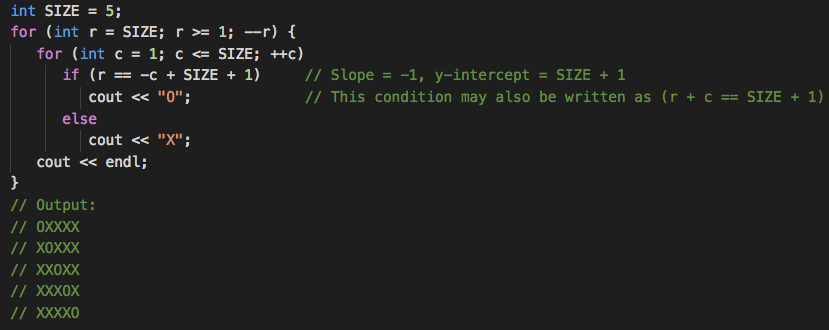
If you're not sure about the y-intercept, draw a numbered grid and double-check a few squares.
Example: Shaded above the diagonal
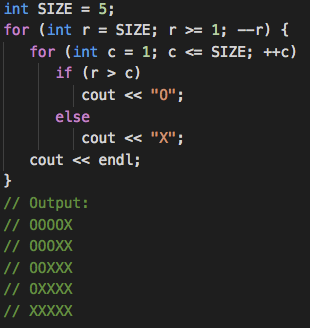
Like in math, use < and > for shaded regions.
Double-check a few squares to make sure you've shaded the correct side.
You can use this strategy to draw triangles (use space for 1 of the 2 characters).
Triangles
Sometimes you don't have to draw the entire rectangle, which may allow you to use a different strategy. In a triangle, the number of characters to print on a particular row depends on the row number.
Example: Lower-left-shaded triangle
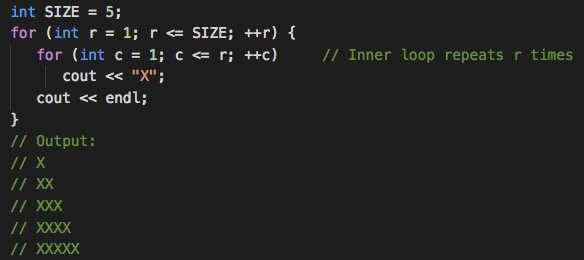
Example: Upper-left-shaded triangle
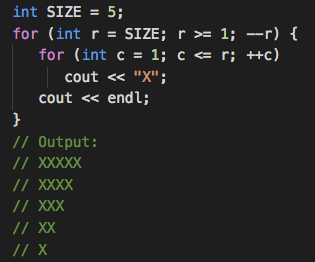
In this example, r counts down so that it coincides with the number of characters in the row.
Example: Pyramid
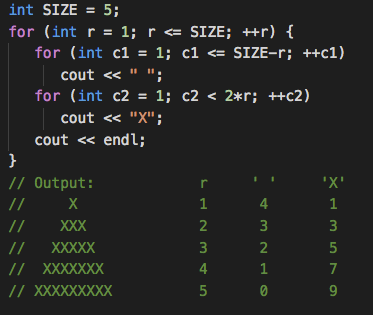
Use 2 inner loops, one for the spaces and one for the Xs.
You can draw a table to figure out how many of each character to print in terms of r.