Conditionals
Statements are executed in order, but some statements, such as conditional statements, cause execution to jump to another point in the code.
Boolean expressions
A boolean expression is an expression that evaluates as true or false. There are two types of operators that evaluate as true/false: relational operators and logical operators. They are listed below, together with arithmetic and assignment.
Precedence | Operator | Description | Associativity |
---|---|---|---|
1 | ! | logical NOT | right-to-left |
2 | * / % | multiplication, division, modulo | left-to-right |
3 | + - | addition, subtraction | left-to-right |
4 | < <= > >= | relational <, ≤, >, ≥ | left-to-right |
5 | == != | relational =, ≠ | left-to-right |
6 | && | logical AND | left-to-right |
7 | || | logical OR | left-to-right |
8 | = | assignment | right-to-left |
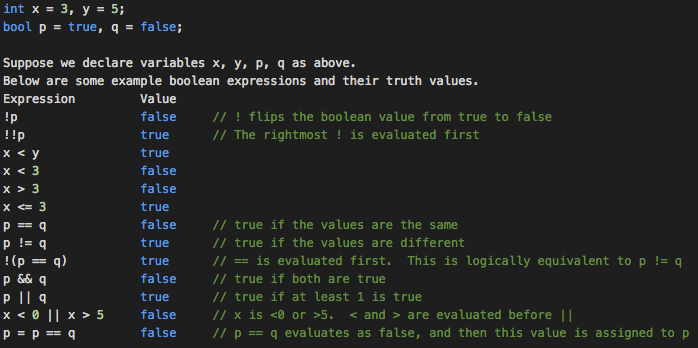
Conditional statements
A conditional statement uses a condition to decide whether or not to execute a block of code. The condition must be a boolean expression. A block is a group of statements surrounded by {}.
if

if-else

- The if-else statement will execute 1 of the 2 blocks, but not both.
- If a block contains only 1 statement, the {} are optional.
- The compiler does not care about indentation, but you should indent anything inside a block to improve readability.
- There is no condition next to the else. An if-else statement only has one condition.
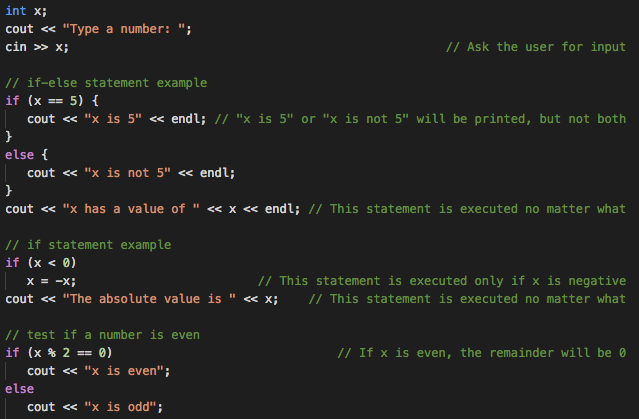
Nested conditionals
A block may include a conditional statement. This allows you to chain if-else statements.
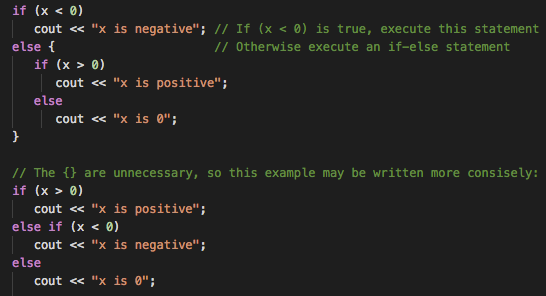
Loops
A loop repeats a block of code as long as a condition is true. If the block contains only 1 statement, the {} are optional.
while

for
Any while-loop can be written as a for-loop. for-loops are often used to repeat a loop a specific number of times. The two loops below are equivalent.

- Initialization is done only once before the first iteration of the loop.
- Increment is done at the end of each iteration of the loop.
- If you omit the condition in a for-loop, it will be evaluated as true.
Incrementing is often done with operators.
- ++i is equivalent to i = i+1 (++i is pre-increment; in the examples below, post-increment would have the same effect)
- --i is equivalent to i = i-1
- i += 3 is equivalent to i = i+3 (there is a compound assignment operator for each arithmetic operator)
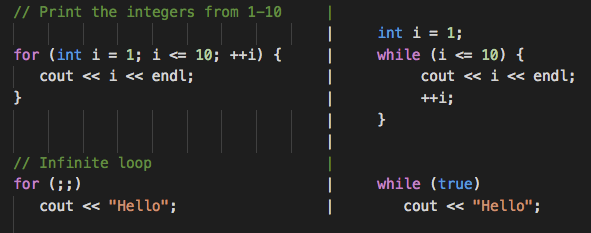
In the first example, each time you evaluate the condition, i has a different value. The 11th time you evaluate the condition, i is 11, so the condition is false and the loop ends.
break and continue
break exits from a loop. continue takes you back to the beginning of a loop. while (true) loops will usually include a break statement to avoid an infinite loop.
Problems involving loops
Digits
Problems involving digits can often be solved with the following strategy. The number of digits in x will be the number of times that the loop repeats, because after removing the last digit that many times, x will be 0.
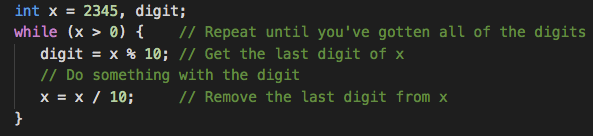