Details
This set of problems and coding challenges are to be done in lab and at home.
All the cpp files from this assignment should be in a folder.
The name of the folder should follow this format:
lastName_firstName_#3
For example, my name is Alex Chen, folder name will be "Chen_Alex_#3"
Failure to follow this naming convention will result in your assignment not being graded.
The folder is to be zipped and submitted on blackboard on the due date.
#1
Write a pattern1.cpp program ask the user for a height. The program prints a triangle of that height.
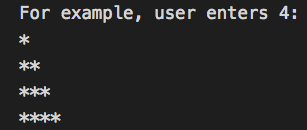
#2
Write a pattern2.cpp program ask the user for a height. The program prints a pyramid of that height.
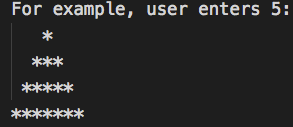
#3
Write a pattern3.cpp program ask the user for a height. The program prints a sideways pyramid of that height.
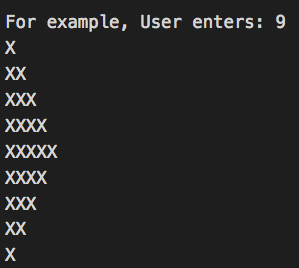
#4
Write a pattern4.cpp program ask the user for a height. The program prints 3 triangles of that height 3 times side by side.
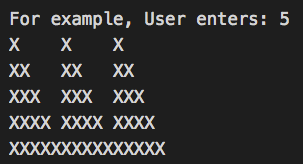
#5
Write a pattern5.cpp program ask the user for a height. The program prints out a blank X against a dark background made of Xs.
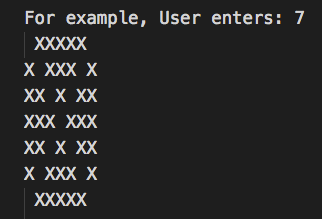
#6
Write a pattern6.cpp program that asks the user for a width w and prints a blank arrow pattern (made of spaces) against a dark background made of Xs.
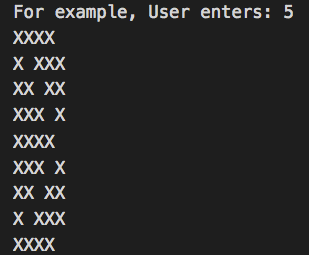
#7
Write a pattern7.cpp program program ask the user for a height. The program prints out an X.
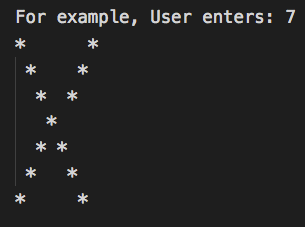
#8
Write a pattern8.cpp program ask the user for a height. The program prints out an X of this form. For example the height is 7.
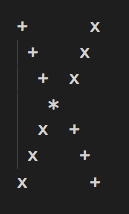
#9
Write a pattern9.cpp program ask the user for a height and width. Print the following pattern.
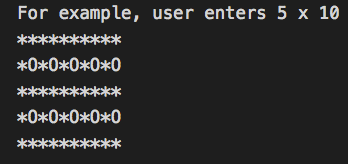
#10
Write a pattern10.cpp program that does the following:
- The program asks the user to enter a positive integer.
- The program reads a value n entered by the user. If the value is not legal, the program should terminate immediately.
- The program prints two copies of a triangular pattern with side n. Each triangle has a horizontal side at the bottom and a vertical side at the right. The second copy should be underneath and to the right of the first.
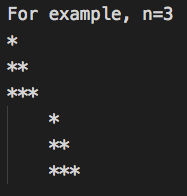