General
The memory needed for a program to run is determined when the program complies. The memory that is needed for the variables declared in a program is allocated before the program is executed.
But there may be cases where the memory cannot be determined until runtime.
For example when the memory needed is dependent on user input. In such cases we need to dynamically allocate memory.
new and new[] operators
Dynamic memory is allocated using the new operator. The syntax is:pointer = new type
This expression is used to allocate memory to contain one single element.
pointer = new type [number_of_numbers]
This expression is used to allocate a block of elements or an array.
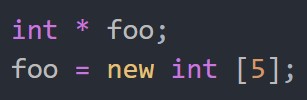
In this example, the program dynamically allocates space for five integers and returns a pointer to the first element in the array. foo is a pointer that now points to a valid block of memory with space for five int elements. See pointers for more info.
It returns a pointer to the beginning of the new block of memory allocated.
The dynamic memory requested by our program is allocated from the memory heap. However, memory is limited, and it can be exhausted.
delete and delete[] operators
One way to prevent memory from being wasted, memory leak, is to free memory up. In most cases, memory allocated dynamically is only needed for a specific period and can be freed up so that the memory becomes available for future use. This is done using the delete operator. The syntax for delete is:delete pointer;
This statement releases the memory of a single element allocated.
delete[] pointer;
This statement releases the memory allocated for arrays.
Related: